vue3使用echarts渲染全球地图+省份地图
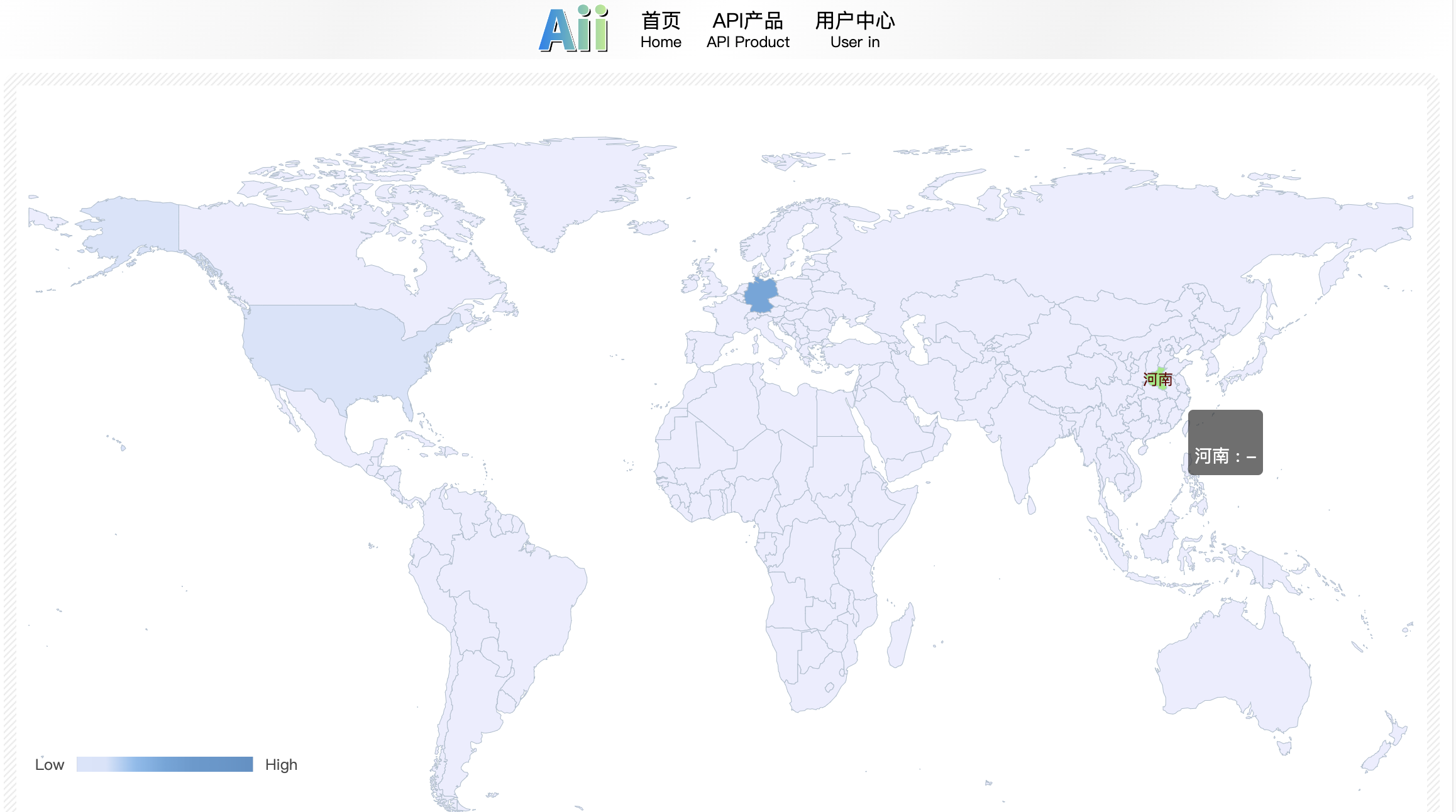
<template> <div ref="map" id="mapxx" style="width:100%; height: 80vh;"></div> </template> <script setup> import { ref, onMounted, } from 'vue'; import world from './map/world.json' //导入世界地图 import china from './map/china.json' //导入中国地图 import * as echarts from 'echarts' onMounted(() => { let data = { data: { map: [ { name: "美国", value: [10, "美国"] }, { name: "中国", value: [100, "中国"], label: '无数据', }, { name: "德国", value: [50, "德国"], label: '无数据', } ] } } drawLine(data) //渲染地图 }) //解码地图 function decodePolygon(coordinate, encodeOffsets, encodeScale) { var result = []; var prevX = encodeOffsets[0]; var prevY = encodeOffsets[1]; for (var i = 0; i < coordinate.length; i += 2) { var x = coordinate.charCodeAt(i) - 64; var y = coordinate.charCodeAt(i + 1) - 64; // ZigZag decoding x = (x >> 1) ^ -(x & 1); y = (y >> 1) ^ -(y & 1); // Delta deocding x += prevX; y += prevY; prevX = x; prevY = y; // Dequantize result.push([x / encodeScale, y / encodeScale]); } return result; } //解码地图 function decode(json) { if (!json.UTF8Encoding) { return json; } var encodeScale = json.UTF8Scale; if (encodeScale == null) { encodeScale = 1024; } var features = json.features; for (var f = 0; f < features.length; f++) { var feature = features[f]; var geometry = feature.geometry; var coordinates = geometry.coordinates; var encodeOffsets = geometry.encodeOffsets; for (var c = 0; c < coordinates.length; c++) { var coordinate = coordinates[c]; if (geometry.type === "Polygon") { coordinates[c] = decodePolygon( coordinate, encodeOffsets[c], encodeScale ); } else if (geometry.type === "MultiPolygon") { for (var c2 = 0; c2 < coordinate.length; c2++) { var polygon = coordinate[c2]; coordinate[c2] = decodePolygon( polygon, encodeOffsets[c][c2], encodeScale ); } } } } // Has been decoded json.UTF8Encoding = false; return json; } //转换英文国名为中文 let nameMap = { Afghanistan: '阿富汗', Singapore: '新加坡', Angola: '安哥拉', Albania: '阿尔巴尼亚', 'United Arab Emirates': '阿联酋', Argentina: '阿根廷', Armenia: '亚美尼亚', 'French Southern and Antarctic Lands': '法属南半球和南极领地', Australia: '澳大利亚', Austria: '奥地利', Azerbaijan: '阿塞拜疆', Burundi: '布隆迪', Belgium: '比利时', Benin: '贝宁', 'Burkina Faso': '布基纳法索', Bangladesh: '孟加拉国', Bulgaria: '保加利亚', 'The Bahamas': '巴哈马', 'Bosnia and Herzegovina': '波斯尼亚和黑塞哥维那', Belarus: '白俄罗斯', Belize: '伯利兹', Bermuda: '百慕大', Bolivia: '玻利维亚', Brazil: '巴西', Brunei: '文莱', Bhutan: '不丹', Botswana: '博茨瓦纳', 'Central African Republic': '中非共和国', Canada: '加拿大', Switzerland: '瑞士', Chile: '智利', China: '中国', 'Ivory Coast': '象牙海岸', Cameroon: '喀麦隆', 'Democratic Republic of the Congo': '刚果民主共和国', 'Republic of the Congo': '刚果共和国', Colombia: '哥伦比亚', 'Costa Rica': '哥斯达黎加', Cuba: '古巴', 'Northern Cyprus': '北塞浦路斯', Cyprus: '塞浦路斯', 'Czech Republic': '捷克共和国', Germany: '德国', Djibouti: '吉布提', Denmark: '丹麦', 'Dominican Republic': '多明尼加共和国', Algeria: '阿尔及利亚', Ecuador: '厄瓜多尔', Egypt: '埃及', Eritrea: '厄立特里亚', Spain: '西班牙', Estonia: '爱沙尼亚', Ethiopia: '埃塞俄比亚', Finland: '芬兰', Fiji: '斐', 'Falkland Islands': '福克兰群岛', France: '法国', Gabon: '加蓬', 'United Kingdom': '英国', Georgia: '格鲁吉亚', Ghana: '加纳', Guinea: '几内亚', Gambia: '冈比亚', 'Guinea Bissau': '几内亚比绍', Greece: '希腊', Greenland: '格陵兰', Guatemala: '危地马拉', 'French Guiana': '法属圭亚那', Guyana: '圭亚那', Honduras: '洪都拉斯', Croatia: '克罗地亚', Haiti: '海地', Hungary: '匈牙利', Indonesia: '印度尼西亚', India: '印度', Ireland: '爱尔兰', Iran: '伊朗', Iraq: '伊拉克', Iceland: '冰岛', Israel: '以色列', Italy: '意大利', Jamaica: '牙买加', Jordan: '约旦', Japan: '日本', Kazakhstan: '哈萨克斯坦', Kenya: '肯尼亚', Kyrgyzstan: '吉尔吉斯斯坦', Cambodia: '柬埔寨', Kosovo: '科索沃', Kuwait: '科威特', Laos: '老挝', Lebanon: '黎巴嫩', Liberia: '利比里亚', Libya: '利比亚', 'Sri Lanka': '斯里兰卡', Lesotho: '莱索托', Lithuania: '立陶宛', Luxembourg: '卢森堡', Latvia: '拉脱维亚', Morocco: '摩洛哥', Moldova: '摩尔多瓦', Madagascar: '马达加斯加', Mexico: '墨西哥', Macedonia: '马其顿', Mali: '马里', Myanmar: '缅甸', Montenegro: '黑山', Mongolia: '蒙古', Mozambique: '莫桑比克', Mauritania: '毛里塔尼亚', Malawi: '马拉维', Malaysia: '马来西亚', Namibia: '纳米比亚', 'New Caledonia': '新喀里多尼亚', Niger: '尼日尔', Nigeria: '尼日利亚', Nicaragua: '尼加拉瓜', Netherlands: '荷兰', Norway: '挪威', Nepal: '尼泊尔', 'New Zealand': '新西兰', Oman: '阿曼', Pakistan: '巴基斯坦', Panama: '巴拿马', Peru: '秘鲁', Philippines: '菲律宾', 'Papua New Guinea': '巴布亚新几内亚', Poland: '波兰', 'Puerto Rico': '波多黎各', 'North Korea': '北朝鲜', Portugal: '葡萄牙', Paraguay: '巴拉圭', Qatar: '卡塔尔', Romania: '罗马尼亚', Russia: '俄罗斯', Rwanda: '卢旺达', 'Western Sahara': '西撒哈拉', 'Saudi Arabia': '沙特阿拉伯', Sudan: '苏丹', 'South Sudan': '南苏丹', Senegal: '塞内加尔', 'Solomon Islands': '所罗门群岛', 'Sierra Leone': '塞拉利昂', 'El Salvador': '萨尔瓦多', Somaliland: '索马里兰', Somalia: '索马里', 'Republic of Serbia': '塞尔维亚', Suriname: '苏里南', Slovakia: '斯洛伐克', Slovenia: '斯洛文尼亚', Sweden: '瑞典', Swaziland: '斯威士兰', Syria: '叙利亚', Chad: '乍得', Togo: '多哥', Thailand: '泰国', Tajikistan: '塔吉克斯坦', Turkmenistan: '土库曼斯坦', 'East Timor': '东帝汶', 'Trinidad and Tobago': '特里尼达和多巴哥', Tunisia: '突尼斯', Turkey: '土耳其', 'United Republic of Tanzania': '坦桑尼亚', Uganda: '乌干达', Ukraine: '乌克兰', Uruguay: '乌拉圭', 'United States': '美国', Uzbekistan: '乌兹别克斯坦', Venezuela: '委内瑞拉', Vietnam: '越南', Vanuatu: '瓦努阿图', 'West Bank': '西岸', Yemen: '也门', 'South Africa': '南非', Zambia: '赞比亚', Korea: '韩国', Tanzania: '坦桑尼亚', Zimbabwe: '津巴布韦', Congo: '刚果', 'Central African Rep.': '中非', Serbia: '塞尔维亚', 'Bosnia and Herz.': '波黑', 'Czech Rep.': '捷克', 'W. Sahara': '西撒哈拉', 'Lao PDR': '老挝', 'Dem.Rep.Korea': '朝鲜', 'Falkland Is.': '福克兰群岛', 'Timor-Leste': '东帝汶', 'Solomon Is.': '所罗门群岛', Palestine: '巴勒斯坦', 'N. Cyprus': '北塞浦路斯', Aland: '奥兰群岛', 'Fr. S. Antarctic Lands': '法属南半球和南极陆地', Mauritius: '毛里求斯', Comoros: '科摩罗', 'Eq. Guinea': '赤道几内亚', 'Guinea-Bissau': '几内亚比绍', 'Dominican Rep.': '多米尼加', 'Saint Lucia': '圣卢西亚', Dominica: '多米尼克', 'Antigua and Barb.': '安提瓜和巴布达', 'U.S. Virgin Is.': '美国原始岛屿', Montserrat: '蒙塞拉特', Grenada: '格林纳达', Barbados: '巴巴多斯', Samoa: '萨摩亚', Bahamas: '巴哈马', 'Cayman Is.': '开曼群岛', 'Faeroe Is.': '法罗群岛', 'IsIe of Man': '马恩岛', Malta: '马耳他共和国', Jersey: '泽西', 'Cape Verde': '佛得角共和国', 'Turks and Caicos Is.': '特克斯和凯科斯群岛', 'St. Vin. and Gren.': '圣文森特和格林纳丁斯' } //渲染地图 const drawLine = (datas) => { // 基于准备好的dom,初始化echarts实例 let myChart = echarts.init(document.getElementById('mapxx')) let worldAndChina = Object.assign({}, world); //深拷贝赋值世界地图数据 // let chinaData = decode(china) //解码中国地图 // let hainanData = decode(hainan) //解码海南地图 worldAndChina.features = worldAndChina.features.concat(china.features); //两者的features合并 // worldAndChina.features = worldAndChina.features.concat(hainanData.features); //其他省份地图两者的features合并 echarts.registerMap("world", worldAndChina);//载入地图数据 myChart.on('click', handleMapClick); //添加点击事件点击地图触发回调handleMapClick函数 myChart.setOption({ baseOption: { visualMap: [{ dimension: 0, left: 10, bottom: 35, orient: 'horizontal', itemWidth: 12, min: 0, max: 100, text: ['High', 'Low'], textStyle: { color: '#4a4a4a' }, inRange: { color: ['#d9e4fb', '#d8e3fa', '#88bbee', '#6ca5dc', '#6199d0', '#5d96cd', '#5890c7'] } }], animationDurationUpdate: 1000, animationEasingUpdate: 'quinticInOut', timeline: { show: false }, backgroundColor: '#ffffff', title: [{ text: 'Total Visits Count', left: 45, bottom: 65, textStyle: { fontSize: 12, color: 'rgba(255,255,255, 0.9)' } }], tooltip: {}, grid: { left: '10%', right: '45%', top: '70%', bottom: 20 }, xAxis: { show: false }, yAxis: { show: false }, nameMap: nameMap, series: [ { id: 'map', type: 'map', mapType: 'world', right: "1%", top: "7%", bottom: "1%", left: 10, roam: true, // 允许缩放和平移 label: { show: false //显示国名 }, // center: [115.97, 29.71], //中心点 itemStyle: { borderColor: '#f11', // 设置省界线颜色 borderWidth: 1.5, // 设置省界线宽度 normal: { areaColor: "#ecedfe", //地图模块未选中颜色 borderColor: "#abc" //地图模块边框 }, emphasis: {//对应的鼠标悬浮效果 show: true, textStyle: { color: "#f11111" }, label: { show: true }, areaColor: "#90ed7d" //选中模块颜色 } }, data: datas.data.map }, ] }, }); window.onresize = () => { myChart.resize(); }; } </script> <style lang="scss" scoped> </style>
文件下载:
1,003 Views